SYNOPSIS
#include <agar/core.h> #include <agar/gui.h>
DESCRIPTION
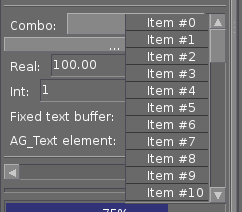

AG_Combo provides the same functionality as AG_UCombo(3), but also adds support for text search and arbitrary text input.
INHERITANCE HIERARCHY ↑
AG_Object(3)-> AG_Widget(3)-> AG_Combo.
INITIALIZATION ↑
AG_Combo * AG_ComboNew (AG_Widget *parent, Uint flags, const char *format, ...)
AG_Combo * AG_ComboNewS (AG_Widget *parent, Uint flags, const char *label)
AG_Combo * AG_ComboNewFn (AG_Widget *parent, Uint flags, const char *label, AG_EventFn fn, const char *fnArgs, ...)
void AG_ComboSizeHint (AG_Combo *combo, const char *text, int nitems)
void AG_ComboSizeHintPixels (AG_Combo *combo, int w, int nitems)
The AG_ComboNew() function allocates, initializes, and attaches a new AG_Combo widget. The string argument specifies an optional text label to be displayed at the left of the textbox. The AG_ComboNewFn() variant sets the "combo-expanded" event handler to fn with optional arguments fnArgs.
Acceptable flags include:
AG_COMBO_POLL | List contents are dynamic (pass the AG_TLIST_POLL flag to the tlist). |
AG_COMBO_ANY_TEXT | Allow user to enter text that does not match any item in the list. |
AG_COMBO_SCROLLTOSEL | Scroll to initial selection if it is not visible. |
AG_COMBO_HFILL | Expand horizontally in parent container. |
AG_COMBO_VFILL | Expand vertically in parent container. |
AG_COMBO_EXPAND | Shorthand for AG_COMBO_HFILL AG_COMBO_VFILL|. |
The AG_ComboSizeHint() function arranges for the AG_Tlist(3) widget displayed on popup to request a size large enough to display the given number of items. The AG_ComboSizeHintPixels() variant specifies the width in number of pixels.
ITEM SELECTION ↑
void AG_ComboSelect (AG_Combo *combo, AG_TlistItem *item)
AG_TlistItem * AG_ComboSelectPointer (AG_Combo *combo, void *ptr)
AG_TlistItem * AG_ComboSelectText (AG_Combo *combo, const char *text)
The AG_ComboSelect() function sets the selection flag on the given item.
The AG_ComboSelectPointer() function selects the first item with a user pointer value matching ptr. Similarly, AG_ComboSelectText() selects the first item with a text string equal to text.
If the AG_COMBO_POLL option is set, both AG_ComboSelectPointer() and AG_ComboSelectText() will raise a tlist-poll event prior to making the selection.
EVENTS ↑
The
AG_Combo widget generates the following events:
combo-selected (AG_TlistItem *item) | An item was selected. |
combo-expanded (void) | The drop-down menu is now visible. May be used to populate list. |
combo-collapsed (void) | The drop-down menu is now hidden. |
combo-text-entry (const char *text) | The AG_COMBO_ANY_TEXT option is set and the user has entered a string text which does not match any item in the list. |
combo-text-unknown (const char *text) | The AG_COMBO_ANY_TEXT flag is not set and the user has entered a string text which does not match any item in the list. |
STRUCTURE DATA ↑
For the
AG_Combo object:
AG_Tlist *list | The AG_Tlist(3) displayed by AG_Combo when expanded, or NULL if collapsed (RO). |
AG_Textbox *tbox | The input AG_Textbox(3) (RO). |
AG_Button *button | The AG_Button(3) which triggers expansion (RO). |
int nVisItems | Initial number of items to show in expanded list. |
EXAMPLES ↑
The following code fragment generates a drop-down menu and reacts to
a selection event by displaying a text dialog:
The following code fragment generates a drop-down menu displaying a tree:
static void ExpandItems(AG_Event *event) { AG_Combo *com = AG_COMBO_SELF(); AG_Tlist *tl = com->list; AG_TlistAdd(tl, NULL, "Foo"); AG_TlistAdd(tl, NULL, "Bar"); } static void SelectItem(AG_Event *event) { AG_TlistItem *item = AG_TLISTITEM_PTR(1); AG_TextMsg(AG_MSG_INFO, "Selected item: %s", item->text); } AG_Combo *com; com = AG_ComboNew(NULL, 0, "My combo: "); AG_SetEvent(com, "combo-expanded", ExpandItems, NULL); AG_SetEvent(com, "combo-selected", SelectItem, NULL);
The following code fragment generates a drop-down menu displaying a tree:
static void ExpandTreeItems(AG_Event *event) { AG_Combo *com = AG_COMBO_SELF(); AG_Tlist *tl = com->list; AG_TlistItem *it; it = AG_TlistAdd(tl, NULL, "Foo"); it->depth = 1; it->flags |= AG_TLIST_HAS_CHILDREN; { it = AG_TlistAdd(tl, NULL, "Bar"); it->depth = 2; it = AG_TlistAdd(tl, NULL, "Baz"); it->depth = 2; { it = AG_TlistAdd(tl, NULL, "Bezo"); it->depth = 3; } } } AG_ComboNewFn(NULL, 0, "My tree: ", ExpandTreeItems, NULL);
SEE ALSO ↑
HISTORY ↑
The
AG_Combo widget first appeared in
Agar 1.0.
As of
Agar 1.6.0,
AG_COMBO_TREE is a deprecated no-op.
"combo-expanded" and "combo-selected" appeared in
Agar 1.7.0.
AG_ComboNewFn() appeared in
Agar 1.7.1.