SYNOPSIS
#include <agar/core.h>
DESCRIPTION
AG_Net provides a cross-platform interface to network sockets, DNS and other
network-related functions.
Available backends include "bsd", "dummy", "winsock1" and "winsock2".
The default backend is selected based on present platform capabilities.
NETWORK ADDRESSES ↑
AG_NetAddrList * AG_NetResolve (const char *hostname, const char *port, Uint flags)
AG_NetAddrList * AG_NetGetIfConfig (void)
AG_NetAddrList * AG_NetAddrListNew (void)
void AG_NetAddrListClear (AG_NetAddrList *list)
void AG_NetAddrListFree (AG_NetAddrList *list)
AG_NetAddr * AG_NetAddrNew (void)
AG_NetAddr * AG_NetAddrDup (const AG_NetAddr *a)
int AG_NetAddrCompare (const AG_NetAddr *a, const AG_NetAddr *b)
int AG_NetAddrIsAny (const AG_NetAddr *a)
const char * AG_NetAddrNumerical (AG_NetAddr *a)
void AG_NetAddrFree (AG_NetAddr *a)
The AG_NetResolve() function looks up a specified host hostname and port number (or service name) port. If the lookup is successful, the results are returned as a list of addresses. Optional flags include:
AG_NET_ADDRCONFIG | Use IPv6 based on host interface status. |
AG_NET_NUMERIC_HOST | Require a hostname in numerical notation. |
AG_NET_NUMERIC_PORT | Require a numerical port number. |
The AG_NetGetIfConfig() function returns the list of addresses associated with local network interfaces (i.e., the list of addresses that would be displayed by ifconfig(8) under Unix). If the call is unsupported on the present platform, the function returns NULL.
The AG_NetAddrList structure stores a list of network addresses. The AG_NetAddrListNew() function returns an empty, newly allocated list, AG_NetAddrListClear() removes all addresses from the given list and AG_NetAddrListFree() releases all resources allocated by the list.
The AG_NetAddr structure stores a single network address and a port number. The AG_NetAddrNew() function returns a newly-allocated address. AG_NetAddrDup() returns a newly-allocated duplicate of a.
AG_NetAddrCompare() evaluates to 0 if a and b represent the same address and port, otherwise a non-zero value (suitable for sorting) is returned. AG_NetAddrIsAny() returns 1 if the address represents "*" (any address).
The AG_NetAddrNumerical() function returns a pointer to an (internally-managed) string buffer containing a numerical representation of the address. The buffer will remains valid until the AG_NetAddr is freed.
The AG_NetAddrFree() routine destroys all resources allocated by a.
SOCKETS ↑
AG_NetSocket * AG_NetSocketNew (enum ag_net_addr_family af, enum ag_net_socket_type type, int proto)
void AG_NetSocketFree (AG_NetSocket *sock)
int AG_NetConnect (AG_NetSocket *sock, const AG_NetAddrList *host)
int AG_NetBind (AG_NetSocket *sock, const AG_NetAddr *addr)
AG_NetSocket * AG_NetAccept (AG_NetSocket *sock)
void AG_NetClose (AG_NetSocket *sock)
int AG_NetRead (AG_NetSocket *sock, void *data, AG_Size size, AG_Size *nRead)
int AG_NetWrite (AG_NetSocket *sock, const void *data, AG_Size size, AG_Size *nWrote)
int AG_NetGetOption (AG_NetSocket *sock, enum ag_net_socket_option opt, void *data)
int AG_NetGetOptionInt (AG_NetSocket *sock, enum ag_net_socket_option opt, int *data)
int AG_NetSetOption (AG_NetSocket *sock, enum ag_net_socket_option opt, const void *data)
int AG_NetSetOptionInt (AG_NetSocket *sock, enum ag_net_socket_option opt, int data)
The AG_NetSocketNew() function creates an endpoint for communication, returning a socket descriptor on success. The af argument indicates the address family of the socket:
AG_NET_AF_NONE | Undefined address family. |
AG_NET_LOCAL | Host-local protocols (e.g., Unix sockets) |
AG_NET_INET4 | Internet version 4 address. |
AG_NET_INET6 | Internet version 6 address. |
The proto argument is an optional protocol number (see protocols(5)). The type argument may be set to:
AG_NET_SOCKET_NONE | Undefined |
AG_NET_STREAM | Stream socket |
AG_NET_DGRAM | Datagram socket |
AG_NET_RAW | Raw-protocol interface |
AG_NET_RDM | Reliably-delivered packet |
AG_NET_SEQPACKET | Sequenced packet stream |
The AG_NetSocketFree() function closes a socket, releasing all associated resources.
The AG_NetConnect() call establishes a connection between sock and a remote host (specified as a list containing one or more addresses to try).
The AG_NetBind() call assigns a local protocol address addr, to the socket sock. The AG_NetAccept() function should be called on a socket that was previously assigned a local address. From the queue of pending connections, AG_NetAccept() extracts the first connection request and returns a newly-created socket for the connection.
The AG_NetClose() routine closes the connection on sock (without destroying the socket).
The AG_NetRead() and AG_NetWrite() routines move data between the socket and a specified buffer data of size bytes. On success, 0 is returned, and the number of bytes successfully read/written is returned in the nRead and nWrite argument (which can be NULL).
The AG_NetGetOption() function returns the current value of the socket option opt into data. AG_NetSetOption() sets the specified socket option to the contents of data. The AG_NetGetOptionInt() and AG_NetSetOptionInt() shorthands accept integer arguments for int socket options. Available socket options are as follows (unless indicated otherwise, int is the data type).
AG_NET_DEBUG | Enable debugging on the socket |
AG_NET_REUSEADDR | Reuse local addresses |
AG_NET_KEEPALIVE | Keep connections alive |
AG_NET_DONTROUTE | Routing bypass for outgoing messages |
AG_NET_BROADCAST | Transmit broadcast messages |
AG_NET_BINDANY | Allow binding to any address |
AG_NET_SNDBUF | Set buffer size for sending |
AG_NET_RCVBUF | Set buffer size for receiving |
AG_NET_SNDLOWAT | Set low watermark for sending |
AG_NET_RCVLOWAT | Set low watermark for receiving |
AG_NET_BACKLOG | Limit on incoming connection backlog |
AG_NET_OOBINLINE | Receive out-of-band data inline (non-portable) |
AG_NET_REUSEPORT | Allow address/port reuse (non-portable) |
AG_NET_TIMESTAMP | Receive datagram timestamps (non-portable) |
AG_NET_NOSIGPIPE | Disable generation of SIGPIPE (non-portable) |
AG_NET_LINGER | Linger on AG_NetClose() up to n seconds if data present (non-portable) |
AG_NET_ACCEPTFILTER | Kernel-based accept filter. The argument is a AG_NetAcceptFilter structure (non-portable) |
The argument to AG_NET_ACCEPTFILTER is defined as follows:
typedef struct ag_net_accept_filter { char name[16]; /* Filter module name */ char arg[240]; /* Argument */ } AG_NetAcceptFilter;
POLLING AND SOCKET SETS ↑
void AG_NetSocketSetInit (AG_NetSocketSet *set)
void AG_NetSocketSetClear (AG_NetSocketSet *set)
void AG_NetSocketSetFree (AG_NetSocketSet *set)
int AG_NetPoll (AG_NetSocketSet *nsInput, AG_NetSocketSet *nsRead, AG_NetSocketSet *nsWrite, AG_NetSocketSet *nsException, Uint32 timeout)
nr ns 0The AG_NetSocketSetInit() function initializes a new socket set. AG_NetSocketSetClear() resets a socket set to the empty set. AG_NetSocketSetFree() releases all resources allocated by a socket set.
The AG_NetPoll() function blocks the execution of the current thread until an event is reported on one or more of the sockets in the nsInput set. Sockets with data available for reading/writing are returned into the nsRead and nsWrite sets. Sockets with other exceptions (such as availability of out-of-band data) are returned into the nsExcept set. If the timeout argument is non-zero, the call will time out in the specified amount of time (given in milliseconds).
STRUCTURE DATA ↑
For the
AG_NetAddr structure:
For the AG_NetSocket structure:
typedef struct ag_net_addr { enum ag_net_addr_family family; /* Address family */ int port; /* Port number (if any) */ union { struct { char *path; /* Unix socket path */ } local; struct { Uint32 addr; /* IPv4 address */ } inet4; struct { Uint8 addr[16]; /* IPv6 address */ } inet6; } data; } AG_NetAddr;
For the AG_NetSocket structure:
enum ag_net_addr_family family | Address family |
enum ag_net_socket_type type | Socket type |
int proto | Socket protocol number |
AG_Mutex lock | Exclusive access lock |
AG_NetAddr *addrLocal | Bound local address |
AG_NetAddr *addrRemote | Connected remote address |
int fd | Socket file descriptor (non-portable) |
void *p | Optional user-defined pointer |
SEE ALSO ↑
HISTORY ↑
The
AG_Net interface first appeared in
Agar 1.5.0.
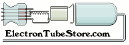